Export gridview to pdf using iTextsharp
In this example i m exporting Gridview populated with SqlDataSource to Pdf using iTextSharp in click event of Button
This is the html source of the page in which i've created a gridview,Sqldatasource and a Button to export GridView to Pdf
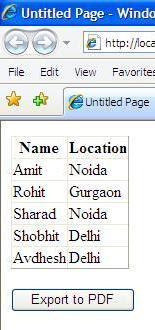
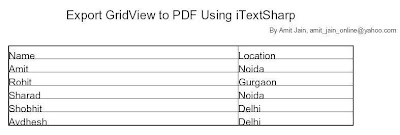
To use iTextSharp , we need to add these namspaces in the code behind and itextsharp.dll in Bin folder of Application
Now in Click event of button i m creating a new HtmlForm and adding the gridview control to this form in code behind , than creating instance of StringWriter class and HtmlTextWriter to write strings and than rendernig these to form created earlier
In next lines of code i m creating a new Document in specified location and opening it for writing
The complete code looks like this
In this example i m exporting Gridview populated with SqlDataSource to Pdf using iTextSharp in click event of Button
This is the html source of the page in which i've created a gridview,Sqldatasource and a Button to export GridView to Pdf
<%@ Page Language="C#" AutoEventWireup="true"
CodeFile="Default.aspx.cs" Inherits="_Default" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN"
"http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml" >
<head runat="server">
<title>Untitled Page</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<asp:GridView ID="GridView1" runat="server"
AutoGenerateColumns="False"
DataSourceID="SqlDataSource1">
<Columns>
<asp:BoundField DataField="Name"
HeaderText="Name"
SortExpression="Name" />
<asp:BoundField DataField="Location"
HeaderText="Location"
SortExpression="Location" />
</Columns>
</asp:GridView>
<asp:SqlDataSource ID="SqlDataSource1"
runat="server"
ConnectionString="<%$ ConnectionStrings:ConnectionString %>"
SelectCommand="SELECT [Name], [Location] FROM [Test]">
</asp:SqlDataSource>
</div>
<br />
<asp:Button ID="btnExport" runat="server"
OnClick="btnExport_Click"
Text="Export to PDF" />
</form>
</body>
</html>
To use iTextSharp , we need to add these namspaces in the code behind and itextsharp.dll in Bin folder of Application
using iTextSharp.text; using iTextSharp.text.pdf; using iTextSharp.text.html; using System.IO; using System.Collections; using System.Net;
Now in Click event of button i m creating a new HtmlForm and adding the gridview control to this form in code behind , than creating instance of StringWriter class and HtmlTextWriter to write strings and than rendernig these to form created earlier
protected void btnExport_Click (object sender, EventArgs e) { HtmlForm form = new HtmlForm(); form.Controls.Add(GridView1); StringWriter sw = new StringWriter(); HtmlTextWriter hTextWriter = new HtmlTextWriter(sw); form.Controls[0].RenderControl(hTextWriter); string html = sw.ToString();
In next lines of code i m creating a new Document in specified location and opening it for writing
Document Doc = new Document(); If u wanna save the pdf in application's root folder in server than use Requesr.PhysicalApplicationPath //PdfWriter.GetInstance //(Doc, new FileStream(Request.PhysicalApplicationPath //+ "\\AmitJain.pdf", FileMode.Create)); And if u wanna save the PDF at users Desktop than use Environment.GetFolderPath(Environment.SpecialFolder.Desktop PdfWriter.GetInstance (Doc, new FileStream(Environment.GetFolderPath (Environment.SpecialFolder.Desktop) + "\\AmitJain.pdf", FileMode.Create)); Doc.Open(); Now i m adding a paragraph to this document to be used as Header by creating a new chuck and adding it to paragraph Chunk c = new Chunk ("Export GridView to PDF Using iTextSharp \n", FontFactory.GetFont("Verdana", 15)); Paragraph p = new Paragraph(); p.Alignment = Element.ALIGN_CENTER; p.Add(c); Chunk chunk1 = new Chunk ("By Amit Jain, amit_jain_online@yahoo.com \n", FontFactory.GetFont("Verdana", 8)); Paragraph p1 = new Paragraph(); p1.Alignment = Element.ALIGN_RIGHT; p1.Add(chunk1); Doc.Add(p); Doc.Add(p1); Now i m reading the html string created above through xmlTextReader and htmlParser to parse html elements System.Xml.XmlTextReader xmlReader = new System.Xml.XmlTextReader(new StringReader(html)); HtmlParser.Parse(Doc, xmlReader); Doc.Close(); string Path = Environment.GetFolderPath (Environment.SpecialFolder.Desktop) + "\\AmitJain.pdf"; ShowPdf(Path);
The complete code looks like this
using System; using System.Data; using System.Configuration; using System.Web; using System.Web.Security; using System.Web.UI; using System.Web.UI.WebControls; using System.Web.UI.WebControls.WebParts; using System.Web.UI.HtmlControls; using iTextSharp.text; using iTextSharp.text.pdf; using iTextSharp.text.html; using System.IO; using System.Collections; using System.Net; public partial class _Default : System.Web.UI.Page { protected void Page_Load(object sender, EventArgs e) { } protected void btnExport_Click(object sender, EventArgs e) { HtmlForm form = new HtmlForm(); form.Controls.Add(GridView1); StringWriter sw = new StringWriter(); HtmlTextWriter hTextWriter = new HtmlTextWriter(sw); form.Controls[0].RenderControl(hTextWriter); string html = sw.ToString(); Document Doc = new Document(); //PdfWriter.GetInstance //(Doc, new FileStream(Request.PhysicalApplicationPath //+ "\\AmitJain.pdf", FileMode.Create)); PdfWriter.GetInstance (Doc, new FileStream(Environment.GetFolderPath (Environment.SpecialFolder.Desktop) + "\\AmitJain.pdf", FileMode.Create)); Doc.Open(); Chunk c = new Chunk ("Export GridView to PDF Using iTextSharp \n", FontFactory.GetFont("Verdana", 15)); Paragraph p = new Paragraph(); p.Alignment = Element.ALIGN_CENTER; p.Add(c); Chunk chunk1 = new Chunk ("By Amit Jain, amit_jain_online@yahoo.com \n", FontFactory.GetFont("Verdana", 8)); Paragraph p1 = new Paragraph(); p1.Alignment = Element.ALIGN_RIGHT; p1.Add(chunk1); Doc.Add(p); Doc.Add(p1); System.Xml.XmlTextReader xmlReader = new System.Xml.XmlTextReader(new StringReader(html)); HtmlParser.Parse(Doc, xmlReader); Doc.Close(); string Path = Environment.GetFolderPath (Environment.SpecialFolder.Desktop) + "\\AmitJain.pdf"; ShowPdf(Path); } private void ShowPdf(string strS) { Response.ClearContent(); Response.ClearHeaders(); Response.ContentType = "application/pdf"; Response.AddHeader ("Content-Disposition","attachment; filename=" + strS); Response.TransmitFile(strS); Response.End(); //Response.WriteFile(strS); Response.Flush(); Response.Clear(); } }
0 comments:
Post a Comment